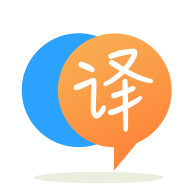
[英]How do I convert a 2D numpy array into a 1D numpy array of 1D numpy arrays?
[英]NumPy: How to check if a tuple is in a 1D numpy array
我在尝试检查 python 元组是否在一维 numpy 数组中时遇到了一些麻烦。 我正在研究一个循环,它将记录图像中存在的所有颜色并将它们存储到一个数组中。 它使用普通列表运行良好,但图像非常大,我认为 NumPy Arrays 会加速循环,因为完成循环需要几分钟。
代码如下所示:
from PIL import Image
import numpy as np
img = Image.open("bg.jpg").convert("RGB")
pixels = img.load()
colors = np.array([])
for h in range(img.size[1]):
for w in range(img.size[0]):
if pixels[w,h] not in colors:
colors = np.append(colors, pixels[w,h])
else:
continue
当我运行它时,我收到以下错误:
DeprecationWarning: elementwise comparison failed; this will raise an error in the future.
if pixels[w,h] in colors:
提前致谢,如果您知道更快的方法,请告诉我。
假设pixels
的形状为(3, w, h)
或(3, h, w)
(即颜色通道沿第一个轴),并假设您所追求的是图像中的独特颜色:
channels = (channel.flatten() for channel in pixels)
colors = set(zip(*channels))
如果你想要一个list
而不是一个集合, colors = list(set(zip(*channels)))
。
你似乎误解了 numpy 派上用场的地方。 一个 numpy 元组数组不会比 Python 元组列表快。 numpy 的速度在矩阵和向量的数值计算中发挥作用。 一个 numpy 元组数组不能利用任何使 numpy 如此快速的东西。
您想要做的根本不适合numpy,并且根本无助于加速您的代码。
我不确定你到底需要什么。 但我希望下一段代码对你有所帮助。
import numpy as np
image = np.arange(75).reshape(5, 5, 3) % 8
# Get the set of unique pixles
pixel_list = image.reshape(-1, 3)
unique_pixels = np.unique(pixel_list, axis = 0)
# Test whether a pixel is in the list of pixels:
i = 0
pixel_in_list = (unique_pixels[i] == pixel_list).all(1).any(0)
# all(1) - all the dimensions (rgb) of the pixels need to match
# any(0) - test if any of the pixels match
# Test whether any of the pixels in the set is in the list of pixels:
compare_all = unique_pixels.reshape(-1, 1, 3) == pixel_list.reshape(1, -1, 3)
pixels_in_list = compare_all.all(2).any()
# all(2) - all the dimensions (rgb) of the pixels need to match
# any() - test if any of the pixelsin the set matches any of the pixels in the list
如何检查元组是否在一维numpy数组中:
>>> colors = [(1,2,3),(4,5,6)]
>>> for x in colors:
print(x)
if isinstance(x, tuple):
print("is tuple")
(1, 2, 3)
is tuple
(4, 5, 6)
is tuple
但是您应该使用列表而不是 numpy 数组。 错误很明显,该功能已被弃用。 这是对标题中提出的问题的回答。
我找到了一种更快的方法来使我的循环在没有 NumPy 的情况下运行得更快,那就是使用sets
,这比使用列表或 NumPy 快得多。 这就是代码现在的样子:
from PIL import Image
img = Image.open("bg.jpg").convert("RGB")
pixels = img.load()
colors = set({})
for h in range(img.size[1]):
for w in range(img.size[0]):
if pixels[w,h] in colors:
continue
else:
colors.add(pixels[w,h])
这解决了我最初的列表太慢而无法循环的问题,并且解决了 NumPy 无法比较元组的第二个问题。 谢谢大家的回复,祝大家有个美好的一天。
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.