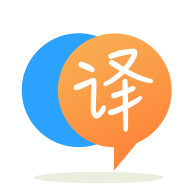
[英]Finding the sum of column of all the rows in a matrix without using numpy
[英]Finding which rows have all elements as zeros in a matrix with numpy
我有一個大的numpy
矩陣M
。 矩陣的某些行的所有元素都為零,我需要獲取這些行的索引。 我正在考慮的天真的方法是遍歷矩陣中的每一行,然后檢查每個元素。 但是,我認為使用numpy
有更好更快的方法來完成此任務。 我希望你能幫忙!
這是一種方法。 我假設已經使用import numpy as np
。
In [20]: a
Out[20]:
array([[0, 1, 0],
[1, 0, 1],
[0, 0, 0],
[1, 1, 0],
[0, 0, 0]])
In [21]: np.where(~a.any(axis=1))[0]
Out[21]: array([2, 4])
這是這個答案的輕微變化: 如何檢查矩陣包含零列?
這是發生了什么:
如果數組中的任何值是“真”,則any
方法返回 True。 非零數被認為是 True,0 被認為是 False。 通過使用參數axis=1
,該方法應用於每一行。 對於示例a
,我們有:
In [32]: a.any(axis=1)
Out[32]: array([ True, True, False, True, False], dtype=bool)
因此,每個值指示相應行是否包含非零值。 ~
運算符是二進制“非”或補碼:
In [33]: ~a.any(axis=1)
Out[33]: array([False, False, True, False, True], dtype=bool)
(給出相同結果的另一種表達式是(a == 0).all(axis=1)
。)
要獲取行索引,我們使用where
函數。 它返回參數為 True 的索引:
In [34]: np.where(~a.any(axis=1))
Out[34]: (array([2, 4]),)
請注意, where
返回了一個包含單個數組的元組。 where
適用於 n 維數組,因此它始終返回一個元組。 我們想要該元組中的單個數組。
In [35]: np.where(~a.any(axis=1))[0]
Out[35]: array([2, 4])
如果元素是int(0)
則接受的答案有效。 如果要查找所有值為 0.0(浮點數)的行,則必須使用np.isclose()
:
print(x)
# output
tensor([[0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.,
0., 0., 0.],
[0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.,
0., 1., 0.],
[0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.,
1., 0., 0.],
[0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.,
0., 0., 0.],
])
np.where(np.all(np.isclose(labels, 0), axis=1))
(array([ 0, 3]),)
注意:這也適用於 PyTorch 張量,當您想找到歸零的多熱編碼向量時,這非常有用。
使用np.sum
解決方案,
如果您想使用閾值,則很有用
a = np.array([[1.0, 1.0, 2.99],
[0.0000054, 0.00000078, 0.00000232],
[0, 0, 0],
[1, 1, 0.0],
[0.0, 0.0, 0.0]])
print(np.where(np.sum(np.abs(a), axis=1)==0)[0])
>>[2 4]
print(np.where(np.sum(np.abs(a), axis=1)<0.0001)[0])
>>[1 2 4]
使用np.prod
檢查行是否包含至少一個零元素
print(np.where(np.prod(a, axis=1)==0)[0])
>>[2 3 4]
a = numpy.array([[10,0],[0,0],[0,10]])
isZero = numpy.all(a == 0, axis=1)
deleteFullZero = a[~numpy.all(a== 0, axis=1)]
#isZero >> [False True False]
#deleteFullZero >> [[10 0][0,10]]
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.