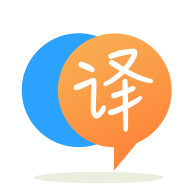
[英]Pandas dataframe - How to sort (alphabetically) column values with value_counts
[英]Change values in pandas dataframe according to value_counts()
我有以下pandas數據幀:
import pandas as pd
from pandas import Series, DataFrame
data = DataFrame({'Qu1': ['apple', 'potato', 'cheese', 'banana', 'cheese', 'banana', 'cheese', 'potato', 'egg'],
'Qu2': ['sausage', 'banana', 'apple', 'apple', 'apple', 'sausage', 'banana', 'banana', 'banana'],
'Qu3': ['apple', 'potato', 'sausage', 'cheese', 'cheese', 'potato', 'cheese', 'potato', 'egg']})
我想更改列中的值Qu1
, Qu2
, Qu3
根據value_counts()
當值數大或等於一定數目
例如,對於Qu1
列
>>> pd.value_counts(data.Qu1) >= 2
cheese True
potato True
banana True
apple False
egg False
我想保留cheese
, potato
, banana
價值,因為每個值至少有兩次出現。
從價值apple
和egg
我想創造價值others
對於列Qu2
沒有變化:
>>> pd.value_counts(data.Qu2) >= 2
banana True
apple True
sausage True
附加的test_data
的最終結果
test_data = DataFrame({'Qu1': ['other', 'potato', 'cheese', 'banana', 'cheese', 'banana', 'cheese', 'potato', 'other'],
'Qu2': ['sausage', 'banana', 'apple', 'apple', 'apple', 'sausage', 'banana', 'banana', 'banana'],
'Qu3': ['other', 'potato', 'other', 'cheese', 'cheese', 'potato', 'cheese', 'potato', 'other']})
謝謝 !
我會創建一個相同形狀的數據框,其中相應的條目是值計數:
data.apply(lambda x: x.map(x.value_counts()))
Out[229]:
Qu1 Qu2 Qu3
0 1 2 1
1 2 4 3
2 3 3 1
3 2 3 3
4 3 3 3
5 2 2 3
6 3 4 3
7 2 4 3
8 1 4 1
並且,使用df.where
的結果返回相應條目小於2的“other”:
data.where(data.apply(lambda x: x.map(x.value_counts()))>=2, "other")
Qu1 Qu2 Qu3
0 other sausage other
1 potato banana potato
2 cheese apple other
3 banana apple cheese
4 cheese apple cheese
5 banana sausage potato
6 cheese banana cheese
7 potato banana potato
8 other banana other
你可以:
value_counts = df.apply(lambda x: x.value_counts())
Qu1 Qu2 Qu3
apple 1.0 3.0 1.0
banana 2.0 4.0 NaN
cheese 3.0 NaN 3.0
egg 1.0 NaN 1.0
potato 2.0 NaN 3.0
sausage NaN 2.0 1.0
然后構建一個包含每列替換的dictionary
:
import cycle
replacements = {}
for col, s in value_counts.items():
if s[s<2].any():
replacements[col] = dict(zip(s[s < 2].index.tolist(), cycle(['other'])))
replacements
{'Qu1': {'egg': 'other', 'apple': 'other'}, 'Qu3': {'egg': 'other', 'apple': 'other', 'sausage': 'other'}}
使用dictionary
替換值:
df.replace(replacements)
Qu1 Qu2 Qu3
0 other sausage other
1 potato banana potato
2 cheese apple other
3 banana apple cheese
4 cheese apple cheese
5 banana sausage potato
6 cheese banana cheese
7 potato banana potato
8 other banana other
或者將循環包含在dictionary
理解中:
from itertools import cycle
df.replace({col: dict(zip(s[s < 2].index.tolist(), cycle(['other']))) for col, s in value_counts.items() if s[s < 2].any()})
但是,這不僅比使用.where
更麻煩,而且速度慢。 使用3,000列進行測試:
df = pd.concat([df for i in range(1000)], axis=1)
<class 'pandas.core.frame.DataFrame'>
RangeIndex: 9 entries, 0 to 8
Columns: 3000 entries, Qu1 to Qu3
dtypes: object(3000)
使用.replace()
:
%%timeit
value_counts = df.apply(lambda x: x.value_counts())
df.replace({col: dict(zip(s[s < 2].index.tolist(), cycle(['other']))) for col, s in value_counts.items() if s[s < 2].any()})
1 loop, best of 3: 4.97 s per loop
vs .where()
:
%%timeit
df.where(df.apply(lambda x: x.map(x.value_counts()))>=2, "other")
1 loop, best of 3: 2.01 s per loop
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.