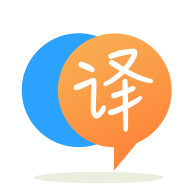
[英]ValueError: shapes (4,1) and (3,1) not aligned: 1 (dim 1) != 3 (dim 0)
[英]ValueError: shapes (1,1) and (4,1) not aligned: 1 (dim 1) != 4 (dim 0)
所以我試圖實現 (a * b) * (M * aT) 但我不斷收到 ValueError。 由於我是 python 和 numpy 函數的新手,所以幫助會很大。 提前致謝。
import numpy.matlib
import numpy as np
def complicated_matrix_function(M, a, b):
ans1 = np.dot(a, b)
ans2 = np.dot(M, a.T)
out = np.dot(ans1, ans2)
return out
M = np.array([[1, 2, 3], [4, 5, 6], [7, 8, 9], [10, 11, 12]])
a = np.array([[1, 1, 0]])
b = np.array([[-1], [2], [5]])
ans = complicated_matrix_function(M, a, b)
print(ans)
print()
print("The size is: ", ans.shape)
錯誤信息是:
ValueError:形狀(1,1)和(4,1)未對齊:1(dim 1)!= 4(dim 0)
錯誤消息告訴您numpy.dot
不知道如何處理 (1x1) 矩陣和 (4x1) 矩陣。 但是,由於在您的公式中您只說要相乘,我假設您只想將標量乘積 (a,b) 中的標量與矩陣向量乘積中的向量相乘(嘛)。 為此,您可以在 python 中簡單地使用*
。
所以你的例子是:
import numpy.matlib
import numpy as np
def complicated_matrix_function(M, a, b):
ans1 = np.dot(a, b)
ans2 = np.dot(M, a.T)
out = ans1 * ans2
return out
M = np.array([[1, 2, 3], [4, 5, 6], [7, 8, 9], [10, 11, 12]])
a = np.array([[1, 1, 0]])
b = np.array([[-1], [2], [5]])
ans = complicated_matrix_function(M, a, b)
print(ans)
print()
print("The size is: ", ans.shape)
導致
[[ 3]
[ 9]
[15]
[21]]
The size is: (4, 1)
筆記
請注意, numpy.dot
會做很多interpretation
來弄清楚你想要做什么。 因此,如果您不需要您的結果大小為 (4,1),您可以將所有內容簡化為:
import numpy.matlib
import numpy as np
def complicated_matrix_function(M, a, b):
ans1 = np.dot(a, b)
ans2 = np.dot(M, a) # no transpose required
out = ans1 * ans2
return out
M = np.array([[1, 2, 3], [4, 5, 6], [7, 8, 9], [10, 11, 12]])
a = np.array([1, 1, 0]) # no extra [] required
b = np.array([-1, 2, 5]) # no extra [] required
ans = complicated_matrix_function(M, a, b)
print(ans)
print()
print("The size is: ", ans.shape)
導致
[ 3 9 15 21]
The size is: (4,)
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.