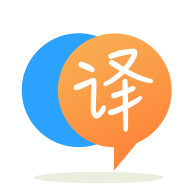
[英]find angle between major axis of ellipse and x-axis of coordinate (help me implement method from paper)
[英]Finding angle between the x-axis and a vector on the unit circle
我有一個函數可以找到單位圓矢量[1,0]
和單位圓上的矢量之間的角度。
import numpy as np
def angle(a):
b = [0,1]
if a[1] >= 0:
return round(np.degrees(np.arccos(np.dot(a, b)/ (np.linalg.norm(a) * np.linalg.norm(b)))), 2)
else:
return 180 + round(np.degrees(np.arccos(np.dot(a, b)/ (np.linalg.norm(a) * np.linalg.norm(b)))), 2)
print(angle(np.array([1,0])))
90.0
print(angle(np.array([-4,2])))
63.43 # This value should be 150
print(angle(np.array([-1,0])))
90.0 # This value should be 180
print(angle(np.array([0,-1])))
360.0 # This value should be 270
a
始終是二維向量?你的 x 向量錯了。 它應該是
b = [1,0]
假設第一個坐標是 x 軸,第二個坐標是 y 軸。 如果你把這個正確的 b 向量,所有的計算都按預期工作。
定義需要輸入的函數的一種方法是將兩者都保留為單獨的參數(這也修復了一些錯誤並簡化了獲取角度值的邏輯):
def angle(x, y):
rad = np.arctan2(y, x)
degrees = np.int(rad*180/np.pi)
if degrees < 0:
degrees = 360 - degrees
return degrees
順便提一下, atan2
輸入順序是y, x
,這很容易混淆。 單獨指定它們的一個優點是您可以幫助避免這種情況。 如果您想將輸入保留為數組,這樣的操作可以幫助您驗證長度:
def angle(a):
if len(a) != 2:
raise IndexError("vector a expected to be length 2")
x = a[0]
y = a[1]
rad = np.arctan2(y, x)
degrees = np.int(rad*180/np.pi)
if degrees < 0:
degrees = 360 - degrees
return degrees
要確定 a 始終是二維向量,您可以檢查它的長度:
if len(a) == 2
。
您還可以通過以下方式檢查它是列表還是元組:
if type(a) in [list, tuple] and len(a) == 2:
編輯:我的壞只是注意到它實際上是 numpy 數組,在這種情況下: if isinstance(x, np.ndarray) and x.shape[0] == 2
編輯 2:來自評論: x.ndim == 2
聽起來更好。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.