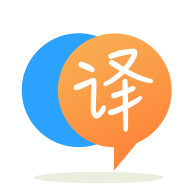
[英]How can I create a color image (RGB), after changing it into a 3 dimensional array of each pixel?
[英]How can I cycle through individual pixels in an image and output the highest RGB valued pixel of each row?
我正在尝试导入图像文件,例如file.bmp,读取图像中每个像素的RGB值,然后将每行的最高RGB值像素(最亮像素)输出到屏幕。 关于如何使用Python做任何建议?
好吧,您可以使用scipy.misc.imread
来读取图像并像这样操作它:
import scipy.misc
file_array = scipy.misc.imread("file.bmp")
def get_brightness(pixel_tuple):
return sum([component*component for component in pixel_tuple])**.5 # distance from (0, 0, 0)
row_maxima = {}
height, width = len(file_array), len(file_array[0])
for y in range(height):
for x in range(width):
pixel = tuple(file_array[y][x]) # casting it to a tuple so it can be stored in the dict
if y in row_maxima and get_brightness(pixel) > row_maxima[y]:
row_maxima[y] = pixel
if y not in row_maxima:
row_maxima[y] = pixel
print row_maxima
你可以在这里充分利用numpy的力量。 请注意,下面的代码输出[0,255]范围内的“亮度”。
#!/bin/env python
import numpy as np
from scipy.misc import imread
#Read in the image
img = imread('/users/solbrig/smooth_test5.png')
#Sum the colors to get brightness
brightness = img.sum(axis=2) / img.shape[2]
#Find the maximum brightness in each row
row_max = np.amax(brightness, axis=1)
print row_max
如果您认为您的图片可能有alpha图层,则可以执行以下操作:
#!/bin/env python
import numpy as np
from scipy.misc import imread
#Read in the image
img = imread('/users/solbrig/smooth_test5.png')
#Pull off alpha layer
if img.shape[2] == 4:
alph = img[:,:,3]/255.0
img = img[:,:,0:3]
else:
alph = np.ones(img.shape[0:1])
#Sum the colors to get brightness
brightness = img.sum(axis=2) / img.shape[2]
brightness *= alph
#Find the maximum brightness in each row
row_max = np.amax(brightness, axis=1)
print row_max
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.