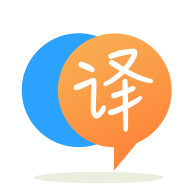
[英]How do you rotate the numbers in an numpy array of shape (n,) or (n,1)?
[英]How do you rotate elements in a 2D numpy array by 'n' units?
x = [[1, 2, 3],
[4, 5, 6],
[7, 8, 9]]
轮换1个单位应该给:
x = [[4, 1, 2],
[7, 5, 3],
[8, 9, 6]]
基本上,我想用'n'单位移动数组中的每个圆形层。
我看了numpy.roll,但无法想出将它用于此用例。 我不能使用像scipy.ndimage.interpolation.rotate这样的图像旋转例程,因为它们会改变形状而不能完全达到预期的效果。
编辑:
对于4 X 4矩阵:
x = [[a, b, c, d],
[e, f, g, h],
[i, j, k, l],
[m, n, o, p]]
轮换1个单位应该给:
x = [[e, a, b, c],
[i, j, f, d],
[m, k, g, h],
[n, o, p, l]]
编辑:
添加一些关于如何适用于任意大小的说明。
对于旋转1个单位的NXN矩阵,外部“环”首先移位1.相同的逻辑递归地跟随剩余的“内部”(N-2)X(N-2)矩阵。
这是一种方法,假设您正在寻找旋转,使得切片的量在切片上是恒定的,其中切片是指从中心向外指向的最外层元素 -
def outer_slice(x):
return np.r_[x[0],x[1:-1,-1],x[-1,:0:-1],x[-1:0:-1,0]]
def rotate_steps(x, shift):
out = np.empty_like(x)
N = x.shape[0]
idx = np.arange(x.size).reshape(x.shape)
for n in range((N+1)//2):
sliced_idx = outer_slice(idx[n:N-n,n:N-n])
out.ravel()[sliced_idx] = np.roll(np.take(x,sliced_idx), shift)
return out
样品运行
案例#1(3 x 3阵列):
In [444]: x
Out[444]:
array([[24, 85, 97],
[51, 33, 11],
[86, 38, 33]])
In [445]: rotate_steps(x,shift=1)
Out[445]:
array([[51, 24, 85],
[86, 33, 97],
[38, 33, 11]])
案例#2(4 x 4阵列):
In [447]: x
Out[447]:
array([[11, 70, 28, 13],
[44, 41, 17, 82],
[47, 32, 89, 25],
[32, 20, 67, 98]])
In [448]: rotate_steps(x,shift=1)
Out[448]:
array([[44, 11, 70, 28],
[47, 32, 41, 13],
[32, 89, 17, 82],
[20, 67, 98, 25]])
In [449]: rotate_steps(x,shift=2)
Out[449]:
array([[47, 44, 11, 70],
[32, 89, 32, 28],
[20, 17, 41, 13],
[67, 98, 25, 82]])
对于3x3阵列,您可以使用np.roll
和ndarray.flat
完成此ndarray.flat
:
>>> x = np.arange(1, 10).reshape((3, 3))
>>> x
array([[1, 2, 3],
[4, 5, 6],
[7, 8, 9]])
>>> i = np.array([0, 1, 2, 5, 8, 7, 6, 3]) # Indices in circular order
>>> x.flat[i]
array([1, 2, 3, 6, 9, 8, 7, 4])
旋转1个单位:
>>> x.flat[i] = np.roll(x.flat[i], 1) # Rotate indices and reassign
>>> x
array([[4, 1, 2],
[7, 5, 3],
[8, 9, 6]])
旋转4个单位:
>>> x.flat[i] = np.roll(x.flat[i], 4)
>>> x
array([[9, 8, 7],
[6, 5, 4],
[3, 2, 1]])
对于4x4的情况,我仍然需要清楚每个“圆圈”是否需要根据它们的长度以不同的“速度”旋转......
你在矩阵上应用了一个排列。 排列通常由向量(i - > p [i])表示,并应用于向量。 如果需要,可以表示矩阵在矩阵中的排列,排列将是对的矩阵,以便(i,j)处的元素移动到m [i,j]。
构建矩阵后,只需要一个简单的循环来应用置换。
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.