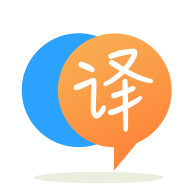
[英]Python - How do I continuously repeat a sequence without a While loop and still be able to stop the sequence at any time
[英]How can I stop these variables from resetting while still being able to loop them?
这是我的代码的一部分:
def dice_game():
dice_window = Tk()
dice_window.geometry('450x450')
player1_score = 0
player2_score = 0
def player1_turn():
player1_num1 = random.randint(1, 6)
player1_num2 = random.randint(1, 6)
player1_score = player1_num1 + player1_num2
player1_total = player1_num1 + player1_num2
if (player1_total % 2) == 0:
player1_score = player1_score + 10
else:
player1_score = player1_score - 5
player1_result = Label(dice_window, text = ( "Player 1 got", player1_num1, "and", player1_num2, "and their total score is:", player1_score))
player1_result.pack()
for x in range(1, 6):
player1_turn()
我尝试将循环放在player1_turn()函数和dice_game()函数中,但结果始终相同。 我如何保持2位玩家的得分而无需在本节每次循环5次时重置它们?
由于您是在player1_turn()
函数的主体内分配player1_score
和player2_score
的,因此它使player1_score & player2_score
成为函数player1_turn()
局部变量。
所以,如果你使用python3
您可以定义player1_score
和player2_score
明确使用视为非本地变量nonlocal
关键字。
该代码将如下所示。
def dice_game():
dice_window = Tk()
dice_window.geometry('450x450')
player1_score = 0
player2_score = 0
def player1_turn():
nonlocal player1_score, player2_score
player1_num1 = random.randint(1, 6)
player1_num2 = random.randint(1, 6)
player1_score = player1_num1 + player1_num2
player1_total = player1_num1 + player1_num2
if (player1_total % 2) == 0:
player1_score = player1_score + 10
else:
player1_score = player1_score - 5
player1_result = Label(dice_window, text = ( "Player 1 got", player1_num1, "and", player1_num2, "and their total score is:", player1_score))
player1_result.pack()
for x in range(1, 6):
player1_turn()
# END
对于python 2,有一种解决方法,您可以将player1_score & player2_score
存储在诸如score = {'player1_score': 0, 'player2_score': 0}
这样的字典中
让我们分解一下。 我可以看到player1_turn()
是嵌套的,因为您想访问dice_window
。 但是有更好的方法!
首先分离功能。 player1_turn
需要一个骰子窗口,看起来要保存的东西是player1_score
,因此我们将其返回。
def player1_turn(dice_window):
player1_num1 = random.randint(1, 6)
player1_num2 = random.randint(1, 6)
player1_score = player1_num1 + player1_num2
player1_total = player1_num1 + player1_num2
if (player1_total % 2) == 0:
player1_score = player1_score + 10
else:
player1_score = player1_score - 5
player1_result = Label(dice_window, text = ( "Player 1 got", player1_num1, "and", player1_num2, "and their total score is:", player1_score))
player1_result.pack()
return player1_score
现在进入游戏:
def dice_game():
dice_window = Tk()
dice_window.geometry('450x450')
# we're not using x, so we should make it clear that this is a loop of 5
scores = []
total = 0
for x in range(5):
player1_score = player1_turn(dice_window)
# now what to do with the score?
scores.append(player1_score) # save it?
print(player1_score) # print it?
total += player1_score # add it up
print(scores)
print(total)
好的,现在的代码在dice_game()函数中具有for循环。 如您所见,您正在将该函数的分数声明为零。 因此,要调用for循环,您需要调用dice_game(),它将得分重新重置为零。
也许您应该尝试在函数dice_game()之外使用for循环,最后,分数将被保留。 或者您可以接受声明
player1_score = 0
player2_score = 0
在函数外部,作为全局变量。
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.