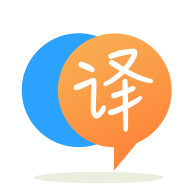
[英]ValueError: Error when checking input: expected flatten_input to have 3 dimensions, but got array with shape (22, 12)
[英]Expected flatten_input to have 3 dimensions, but got array with shape
我正在关注tensorflow的基本分类教程。 由于代理原因,我必须离线使用数据集。 因此,我使用的是 mnist 数据集,而不是使用 fashion_mnist 数据库。
from __future__ import absolute_import, division,
print_function, unicode_literals
# TensorFlow and tf.keras
import tensorflow as tf
from tensorflow import keras
# Helper libraries
import numpy as np
import matplotlib.pyplot as plt
from tensorflow.keras.layers import Flatten, Dense
# Noting class names
class_names = ['Zero', 'One', 'Two', 'Three', 'Four', 'Five', 'Six', 'Seven', 'Eight', 'Nine']
# Load dataset
mnist = keras.datasets.mnist
path = 'C:/projects/VirtualEnvironment/MyScripts/load/mnist.npz'
(train_x, train_y), (test_x, test_y) = mnist.load_data(path)
# Scale, so that training and testing set is preprocessed in the same way
train_x = train_x / 255.0
test_x = test_y / 255.0
train_y = tf.expand_dims(train_y, axis = -1)
test_y = tf.expand_dims(test_y, axis = -1)
#Build the model
#1. Setup the layers
model = keras.Sequential()
model.add(Flatten(input_shape = (28, 28)))
model.add(Dense(128, activation=tf.nn.relu))
model.add(Dense(10, activation=tf.nn.softmax))
#2. Compile the model
model.compile(optimizer='adam',
loss='sparse_categorical_crossentropy',
metrics=['accuracy'])
# Train the model
model.fit(train_x, train_y, epochs=1)
print("Finished Training")
# Evaluate how the model performs on the test dataset
test_loss, test_acc = model.evaluate(test_x, test_y, verbose=2)
我收到以下错误: ValueError: Error when checking input: expected flatten_input to have 3 dimensions, but got array with shape (10000, 1).
我对 tensorflow 知之甚少,所以如果有人可以指导我找到有用的网页,或者可以向我解释错误的含义,我将非常感激
这对我有用:
(train_x, train_y), (test_x, test_y) = tf.keras.datasets.mnist.load_data()
# Scale, so that training and testing set is preprocessed in the same way
train_x = train_x / 255.0
test_x = test_x / 255.0
model = tf.keras.Sequential()
model.add(tf.keras.layers.Flatten(input_shape = (28, 28)))
model.add(tf.keras.layers.Dense(128, activation=tf.nn.relu))
model.add(tf.keras.layers.Dense(10, activation=tf.nn.softmax))
#2. Compile the model
model.compile(optimizer='adam',
loss='sparse_categorical_crossentropy',
metrics=['accuracy'])
# Train the model
model.fit(train_x, train_y, epochs=1)
# Evaluate the model
test_loss, test_acc = model.evaluate(test_x, test_y, verbose=2)
您收到的错误意味着您在代码中的某处错误地重新调整了输入。
这是由您的代码中的拼写错误引起的
改变这个
test_x = test_y / 255.0
至
test_x = test_x / 255.0
我不确定数组形状问题,但是我可以帮助您解决代理问题,以便您可以正确下载数据集。 假设您从 IT 部门清楚了解如何使用工具,您可以通过在终端级别导出来设置 pip 代理:
假设您的登录凭据是 COMPANY\username
export http_proxy=http://COMPANY%5Cusername:password@proxy_ip:proxy_port
export https_proxy=http://COMPANY%5Cusername:password@proxy_ip:proxy_port
如果您使用的是 conda 环境,请使用 C:\Users\username 处的 .condarc 并编辑为:
channels:
- defaults
# Show channel URLs when displaying what is going to be downloaded and
# in 'conda list'. The default is False.
show_channel_urls: True
allow_other_channels: True
proxy_servers:
http: http://COMPANY\username:password@proxy_ip:proxy_port
https: https://COMPANY\username:password@proxy_ip:proxy_port
ssl_verify: False
希望能帮助到你。 为了调试数组形状,我建议您在扩展尺寸后使用 train_y.shape() 和 train_x.shape() 打印 train_y 和 train_x 形状。 该错误指定它得到一个 10000D object 值 1 不应该是这种情况。
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.