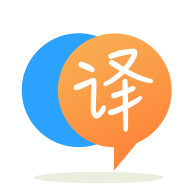
[英]Fastest way to populate a matrix with a function on pairs of elements in two numpy vectors?
[英]Fastest way to find all unique pairs of (nearly) parallel 3d vectors from N vectors in Numpy
我有一个N = 10000
3d 向量的大矩阵。 为简化起见,我将在这里使用一个 10 x 3 矩阵作为示例:
import numpy as np
A = np.array([[1.2, 2.3, 0.8],
[3.2, 2.1, 0.5],
[0.8, 4.4, 4.4],
[-0.2, -1.1, -1.1],
[2.4, 4.6, 1.6],
[0.5, 0.96, 0.33],
[1.1, 2.2, 3.3],
[-2.2, -4.41, -6.62],
[3.4, 5.5, 3.8],
[-5.1, -28., -28.1]])
我想找到几乎彼此平行的所有唯一向量对。 需要使用公差测量,我想获得所有唯一的行索引对(无论顺序如何)。 我设法编写了以下代码:
def all_parallel_pairs(A, tol=0.1):
res = set()
for i, v1 in enumerate(A):
for j, v2 in enumerate(A):
if i == j:
continue
norm = np.linalg.norm(np.cross(v1, v2))
if np.isclose(norm, 0., rtol=0, atol=tol):
res.add(tuple(sorted([i, j])))
return np.array(list(res))
print(all_parallel_pairs(A, tol=0.1))
out[1]: [[0 4]
[2 3]
[6 7]
[4 5]
[0 5]]
但是,由于我使用了两个 for 循环,因此当N
很大时它会变慢。 我觉得应该有更有效和 Numpyic 的方法来做到这一点。 有什么建议么?
请注意,function np.cross
接收来自文档的向量数组:
返回两个(数组)向量的叉积。
因此,一种方法是使用 numpy 高级索引来找到需要计算叉积的正确向量:
# generate the i, j indices (note that only the upper triangular matrices of indices is needed)
rows, cols = np.triu_indices(A.shape[0], 1)
# find the cross products using numpy indexing on A, and the np.cross can take array of vectors
cross = np.cross(A[rows], A[cols])
# find the values that are close to 0
arg = np.argwhere(np.isclose(0, (cross * cross).sum(axis=1) ** 0.5, rtol=0, atol=0.1))
# get the i, j indices where is 0
res = np.hstack([rows[arg], cols[arg]])
print(res)
Output
[[0 4]
[0 5]
[2 3]
[4 5]
[6 7]]
表达方式:
(cross * cross).sum(axis=1) ** 0.5
是在向量数组上应用np.linalg.norm
的更快替代方法。
作为对Dani Masejo answer的改进更新,您可以使用 GPU_aided 或 TPU_aided 库,例如JAX :
from jax import jit
@jit
def test_jit():
rows, cols = np.triu_indices(A.shape[0], 1)
cross = np.cross(A[rows], A[cols])
arg = np.argwhere(np.isclose(0, (cross * cross).sum(axis=1) ** 0.5, rtol=0, atol=0.1))
res = np.hstack([rows[arg], cols[arg]])
return res
print(test_jit())
使用 google colab TPU 运行时的结果如下:
100 loops, best of 5: 12.2 ms per loop # the question code
100 loops, best of 5: 152 µs per loop # Dani Masejo code
100 loops, best of 5: 81.5 µs per loop # using jax library
当数据量增加时差异会很大。
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.