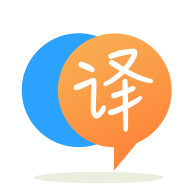
[英]How can I limit the rotation on the Y axis so that the player can't continuously spin the camera in Unity
[英]How can I limit the rotation on the Y axis so the player cant spin the camera 360 in Unity?
我有一個即將到來的項目,我必須在周一提交,這是我必須解決的最后一個錯誤。 如果有人幫助我並教我如何應用軸限制器,那就太好了。 提前謝謝大家。 問題是相機可以旋轉 360 度,這是我正在從事的項目唯一留下的錯誤。
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.AI;
public class CharController_Motor : MonoBehaviour {
private float speed = 10.0f;
public float walkSpeed=7.0f;
public float runSpeed = 12.0f;
public float sensitivity = 30.0f;
public float WaterHeight = 15.5f;
CharacterController character;
private GameObject cam;
private NavMeshAgent nav;
private bool AIActive = true;
public GameObject cam1;
public GameObject cam2;//Nightvision
//private bool NightVision = false;
float moveFB, moveLR;
float rotX, rotY;
float gravity = -9.8f;
public float Stamina = 10f;
public float MaxStamina = 10f;
public int DecayRate = 1;
public float RefillRate=0.25f;
public GameObject LightBreathing;
public GameObject HeavyBreathing;
private bool LightBreath = false;
private bool HeavyBreath = false;
public GameObject LowHealthSound;
public GameObject PlayerDeath;
public GameObject ChaseMusic;
void Start(){
//LockCursor ();
character = GetComponent<CharacterController> ();
SaveScript.PlayerHealth = 30;
sensitivity = sensitivity * 1.5f;
speed = walkSpeed;
Cursor.visible = false;
cam = cam1;
cam2.gameObject.SetActive(false);
LightBreathing.gameObject.SetActive(false);
HeavyBreathing.gameObject.SetActive(false);
nav = GetComponent<NavMeshAgent>();
AIActive = true;
LowHealthSound.gameObject.SetActive(false);
PlayerDeath.gameObject.SetActive(false);
ChaseMusic.gameObject.SetActive(false);
}
void CheckForWaterHeight(){
if (transform.position.y < WaterHeight) {
gravity = 0f;
} else {
gravity = -9.8f;
}
}
void Update(){
if (LightBreath == false)
{
if (Stamina < 3)
{
LightBreathing.gameObject.SetActive(true);
HeavyBreathing.gameObject.SetActive(false);
LightBreath = true;
}
}
if (LightBreath == true)
{
if (Stamina > 3)
{
LightBreathing.gameObject.SetActive(false);
HeavyBreathing.gameObject.SetActive(false);
LightBreath = false;
}
}
if (HeavyBreath == false)
{
if (Stamina == 0)
{
LightBreathing.gameObject.SetActive(false);
HeavyBreathing.gameObject.SetActive(true);
HeavyBreath = true;
}
}
if (HeavyBreath == true)
{
if (Stamina > 0)
{
LightBreathing.gameObject.SetActive(false);
HeavyBreathing.gameObject.SetActive(false);
HeavyBreath = false;
}
}
if (Stamina > 0)
{
if (Input.GetButton("Run"))
{
speed = runSpeed;
Stamina = Stamina - DecayRate * Time.deltaTime;
if (Stamina < 0)
{
Stamina = 0;
}
}
else
{
speed = walkSpeed;
Stamina = Stamina + RefillRate * Time.deltaTime;
if (Stamina > MaxStamina)
{
Stamina = MaxStamina;
}
}
}
if (Stamina == 0)
{
speed = walkSpeed;
StartCoroutine(StaminaRefill());
}
if (SaveScript.PlayerHealth < 5)
{
LowHealthSound.gameObject.SetActive(true);
}
if (SaveScript.PlayerHealth > 4)
{
LowHealthSound.gameObject.SetActive(false);
}
if(SaveScript.PlayerHealth <= 0)
{
PlayerDeath.gameObject.SetActive(true);
}
if (AIActive == true)
{
nav.enabled = true;
}
else
{
nav.enabled = false;
}
if (SaveScript.NightVision==false)
{
cam = cam1;
cam2.gameObject.SetActive(false);//Deactivate NightVision
SaveScript.NightVision = false;
}
if (Input.GetKeyDown(KeyCode.N))
{
if (SaveScript.NightVision == false)
{
cam2.gameObject.SetActive(true);//Activate NightVision
cam = cam2;
SaveScript.NightVision = true;
}
else
{
cam = cam1;
cam2.gameObject.SetActive(false);//Deactivate NightVision
SaveScript.NightVision = false;
}
}
moveFB = Input.GetAxis ("Horizontal") * speed;
moveLR = Input.GetAxis ("Vertical") * speed;
rotX = Input.GetAxis ("Mouse X") * sensitivity;
rotY = Input.GetAxis ("Mouse Y") * sensitivity;
//rotX = Input.GetKey (KeyCode.Joystick1Button4);
//rotY = Input.GetKey (KeyCode.Joystick1Button5);
CheckForWaterHeight ();
Vector3 movement = new Vector3 (moveFB, gravity, moveLR);
CameraRotation(cam, rotX, rotY);
movement = transform.rotation * movement;
character.Move (movement * Time.deltaTime);
}
void CameraRotation(GameObject cam, float rotX, float rotY){
transform.Rotate (0, rotX * Time.deltaTime, 0);
cam.transform.Rotate (-rotY * Time.deltaTime, 0, 0);
}
private void OnTriggerEnter(Collider other)
{
if (other.gameObject.CompareTag("Water"))
{
AIActive = false;
}
}
private void OnTriggerExit(Collider other)
{
if (other.gameObject.CompareTag("Water"))
{
AIActive = true;
}
}
IEnumerator StaminaRefill()
{
yield return new WaitForSeconds(MaxStamina);//Wait 10 seconds before setting stamina to max
if (Stamina == 0)
{
Stamina = MaxStamina;
}
}
}
已編輯。 如果我理解正確 - -rotY * Time.deltaTime
使您的相機在 Y 軸上旋轉。 您可以像這樣將 rotY 值限制在 90 到 180 之間:
void CameraRotation(GameObject cam, float rotX, float rotY){
if(rotY > 180f /*Some value to limit*/)
{
rotY = 180f;
}
else if(rotY < 90f /*Some value to limit*/ )
{
rotY = 90f;
}
transform.Rotate (0, rotX * Time.deltaTime, 0);
cam.transform.Rotate (-rotY * Time.deltaTime, 0, 0);
}
而且,我認為 transform.Rotate 使用 x,y,z 值。 為什么你的cam.transform.Rotate
在它的 x 軸上以-rotY
(y) 值旋轉?
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.