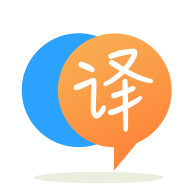
[英]How can I get pixel coordinates of certain color(RGB) of this image using python?
[英]How to get pixel coordinates if I know color(RGB)?
我使用 Python、opencv 和 PIL。
image = cv2.imread('image.jpg')
color = (235, 187, 7)
如果我知道像素颜色,如何获得像素坐标(x,y)?
这是一个 numpythonic 解决方案。 Numpy 库尽可能加快操作速度。
color = (235, 187, 7)
indices = np.where(img == color)
现在indices
返回如下内容:
(array([ 81, 81, 81, ..., 304, 304, 304], dtype=int64),
array([317, 317, 317, ..., 520, 520, 520], dtype=int64),
array([0, 1, 2, ..., 0, 1, 2], dtype=int64))
coordinates = zip(indices[0], indices[1])
set()
方法来完成。 unique_coordinates = list(set(list(coordinates)))
尝试类似:
color = (235, 187, 7)
im = Image.open('image.gif')
rgb_im = im.convert('RGB')
for x in range(rgb_im.size()[0]):
for y in range(rgb_im.size()[1]):
r, g, b = rgb_im.getpixel((x, y))
if (r,g,b) == colour:
print(f"Found {colour} at {x},{y}!")
但是getpixel 可能很慢,所以看看使用像素访问对象。
另请注意,返回的值可能取决于图像类型。 例如,使用pix[1, 1]
返回单个值pix[1, 1]
因为 GIF 像素引用 GIF 调色板中的 256 个值之一。
另请参阅此 SO 帖子: GIF 和 JPEG 的 Python 和 PIL 像素值不同,此PIL 参考页面包含有关convert()
函数的更多信息。
顺便说一下,您的代码对于.jpg
图像可以正常工作。
您可以使用以下内容:
import numpy as np
# for color image
color = (75, 75, 75)
pixels = np.argwhere(img == color)
输出(它重复相同的坐标三次(颜色数)):
[[ 0 28 0]
[ 0 28 1]
[ 0 28 2]
[ 0 54 0]
[ 0 54 1]
[ 0 54 2]
................]
为避免它执行以下操作(抱歉代码可读性):
pixels = pixels[::3][:, [0, 1]]
输出:
[[ 0 28]
[ 0 54]
...........]
对于灰度图像,它看起来更好:
color = (75)
pixels = np.argwhere(img == color)
输出:
[[ 0 28]
[ 0 54]
...........]
import PIL #The reason I use PIL and not opencv is that I find pillow
#(which is imported with 'PIL') a very useful library for image editing.
image = PIL.Image.open('Name_image') #the image is opened and named image
f = image.load() #I'm not sure what the load() operation exactly does, but it
# is necesarry.
color = (235, 187, 7) # the Red Green Blue values that you want to find the
#coordinates of
PixelCoordinates = [] # List in which all pixel coordinates that match
#requirements will be added.
#The lines of code below check for each pixel in the image if the RGB-values
# are equal to (235, 187, 7)
for x in image.size[0]:
for y in image.size[1]:
if f[x,y] == color:
PixelCoordinates.append([x,y])
这是仅使用 cv2 库的解决方案
import cv2
blue = int(input("Enter blue value: "))
green = int(input("Enter green value: "))
red = int(input("Enter red value: "))
path = str(input("Enter image path with image extension:"))
img = cv2.imread(path)
img= cv2.resize(img,(150,150))
x,y,z = img.shape
for i in range(x):
for j in range(y):
if img[i,j,0]==blue & img[i,j,1]==green & img[i,j,1]==red:
print("Found color at ",i,j)
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.