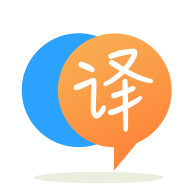
[英]tensorflow code error ValueError: Exception encountered when calling layer "sequential" (type Sequential)
[英]ValueError: Exception encountered when calling layer "sequential_5" (type Sequential)
我正在学习这门课程: TensorFlow 2022 年开发人员证书:从零到精通
这是以下代码:
# Set random seed
tf.random.set_seed(42)
# Create some regression data
X_regression = np.arange(0, 1000, 5)
y_regression = np.arange(100, 1100, 5)
# Split it into training and test sets
X_reg_train = X_regression[:150]
X_reg_test = X_regression[150:]
y_reg_train = y_regression[:150]
y_reg_test = y_regression[150:]
# Setup random seed
tf.random.set_seed(42)
# Recreate the model
model_3 = tf.keras.Sequential([
tf.keras.layers.Dense(100),
tf.keras.layers.Dense(10),
tf.keras.layers.Dense(1)
])
# Change the loss and metrics of our compiled model
model_3.compile(loss=tf.keras.losses.mae, # change the loss function to be regression-specific
optimizer=tf.keras.optimizers.Adam(),
metrics=['mae']) # change the metric to be regression-specific
# Fit the recompiled model
model_3.fit(X_reg_train, y_reg_train, epochs=100)
为什么会出现以下错误,我该如何解决?
您只需要为您的数据添加第二个维度。 它必须是 (batch_size, features)。 您可以使用np.expand_dims
将输入从 (batch_size,) 更改为 (batch_size, features):
import tensorflow as tf
import numpy as np
tf.random.set_seed(42)
# Create some regression data
X_regression = np.expand_dims(np.arange(0, 1000, 5), axis=1)
y_regression = np.expand_dims(np.arange(100, 1100, 5), axis=1)
# Split it into training and test sets
X_reg_train = X_regression[:150]
X_reg_test = X_regression[150:]
y_reg_train = y_regression[:150]
y_reg_test = y_regression[150:]
tf.random.set_seed(42)
# Recreate the model
model_3 = tf.keras.Sequential([
tf.keras.layers.Dense(100),
tf.keras.layers.Dense(10),
tf.keras.layers.Dense(1)
])
# Change the loss and metrics of our compiled model
model_3.compile(loss=tf.keras.losses.mae, # change the loss function to be regression-specific
optimizer=tf.keras.optimizers.Adam(),
metrics=['mae']) # change the metric to be regression-specific
# Fit the recompiled model
model_3.fit(X_reg_train, y_reg_train, epochs=100)
解决此问题的简单方法是使用 function 将 numpy 数组重塑为二维数组.reshape(-1,1)
您可以得到 m
# Set random seed
tf.random.set_seed(42)
# Create some regression data
X_regression = np.arange(0, 1000, 5).reshape(-1,1)
y_regression = np.arange(100, 1100, 5).reshape(-1,1)
# Split it into training and test sets
X_reg_train = X_regression[:150]
X_reg_test = X_regression[150:]
y_reg_train = y_regression[:150]
y_reg_test = y_regression[150:]
# Setup random seed
tf.random.set_seed(42)
# Recreate the model
model_3 = tf.keras.Sequential([
tf.keras.layers.Dense(100),
tf.keras.layers.Dense(10),
tf.keras.layers.Dense(1)
])
# Change the loss and metrics of our compiled model
model_3.compile(loss=tf.keras.losses.mae, # change the loss function to be regression-specific
optimizer=tf.keras.optimizers.Adam(),
metrics=['mae']) # change the metric to be regression-specific
# Fit the recompiled model
model_3.fit(X_reg_train, y_reg_train, epochs=100)
Output
Epoch 1/100
5/5 [==============================] - 1s 3ms/step - loss: 248.2155 - mae: 248.2155
Epoch 2/100
5/5 [==============================] - 0s 4ms/step - loss: 138.9005 - mae: 138.9005
Epoch 3/100
5/5 [==============================] - 0s 4ms/step - loss: 53.1039 - mae: 53.1039
Epoch 4/100
5/5 [==============================] - 0s 4ms/step - loss: 73.5170 - mae: 73.5170
Epoch 5/100
5/5 [==============================] - 0s 4ms/step - loss: 71.2358 - mae: 71.2358
Epoch 6/100
5/5 [==============================] - 0s 3ms/step - loss: 47.0040 - mae: 47.0040
Epoch 7/100
5/5 [==============================] - 0s 3ms/step - loss: 45.9386 - mae: 45.9386
Epoch 8/100
5/5 [==============================] - 0s 4ms/step - loss: 42.3638 - mae: 42.3638
Epoch 9/100
5/5 [==============================] - 0s 3ms/step - loss: 43.6831 - mae: 43.6831
Epoch 10/100
5/5 [==============================] - 0s 3ms/step - loss: 42.6198 - mae: 42.6198
Epoch 11/100
5/5 [==============================] - 0s 4ms/step - loss: 42.4797 - mae: 42.4797
Epoch 12/100
5/5 [==============================] - 0s 4ms/step - loss: 41.5537 - mae: 41.5537
Epoch 13/100
5/5 [==============================] - 0s 4ms/step - loss: 42.0972 - mae: 42.0972
Epoch 14/100
5/5 [==============================] - 0s 3ms/step - loss: 41.8647 - mae: 41.8647
Epoch 15/100
5/5 [==============================] - 0s 4ms/step - loss: 41.5342 - mae: 41.5342
Epoch 16/100
5/5 [==============================] - 0s 4ms/step - loss: 41.4028 - mae: 41.4028
Epoch 17/100
5/5 [==============================] - 0s 3ms/step - loss: 41.6887 - mae: 41.6887
Epoch 18/100
5/5 [==============================] - 0s 4ms/step - loss: 41.6137 - mae: 41.6137
Epoch 19/100
5/5 [==============================] - 0s 3ms/step - loss: 41.2796 - mae: 41.2796
Epoch 20/100
5/5 [==============================] - 0s 4ms/step - loss: 41.1947 - mae: 41.1947
Epoch 21/100
5/5 [==============================] - 0s 4ms/step - loss: 41.2130 - mae: 41.2130
Epoch 22/100
5/5 [==============================] - 0s 4ms/step - loss: 41.0893 - mae: 41.0893
Epoch 23/100
5/5 [==============================] - 0s 4ms/step - loss: 41.2019 - mae: 41.2019
Epoch 24/100
5/5 [==============================] - 0s 4ms/step - loss: 40.9989 - mae: 40.9989
Epoch 25/100
5/5 [==============================] - 0s 5ms/step - loss: 41.0131 - mae: 41.0131
Epoch 26/100
5/5 [==============================] - 0s 4ms/step - loss: 41.0654 - mae: 41.0654
Epoch 27/100
5/5 [==============================] - 0s 3ms/step - loss: 40.8764 - mae: 40.8764
Epoch 28/100
5/5 [==============================] - 0s 4ms/step - loss: 41.0545 - mae: 41.0545
Epoch 29/100
5/5 [==============================] - 0s 5ms/step - loss: 41.0480 - mae: 41.0480
Epoch 30/100
5/5 [==============================] - 0s 3ms/step - loss: 40.8807 - mae: 40.8807
Epoch 31/100
5/5 [==============================] - 0s 4ms/step - loss: 41.2695 - mae: 41.2695
Epoch 32/100
5/5 [==============================] - 0s 4ms/step - loss: 40.9949 - mae: 40.9949
Epoch 33/100
5/5 [==============================] - 0s 3ms/step - loss: 41.0760 - mae: 41.0760
Epoch 34/100
5/5 [==============================] - 0s 3ms/step - loss: 41.2471 - mae: 41.2471
Epoch 35/100
5/5 [==============================] - 0s 5ms/step - loss: 40.6102 - mae: 40.6102
Epoch 36/100
5/5 [==============================] - 0s 4ms/step - loss: 41.1093 - mae: 41.1093
Epoch 37/100
5/5 [==============================] - 0s 3ms/step - loss: 40.8191 - mae: 40.8191
Epoch 38/100
5/5 [==============================] - 0s 4ms/step - loss: 40.2485 - mae: 40.2485
Epoch 39/100
5/5 [==============================] - 0s 3ms/step - loss: 41.0625 - mae: 41.0625
Epoch 40/100
5/5 [==============================] - 0s 3ms/step - loss: 40.5311 - mae: 40.5311
Epoch 41/100
5/5 [==============================] - 0s 3ms/step - loss: 40.5497 - mae: 40.5497
Epoch 42/100
5/5 [==============================] - 0s 4ms/step - loss: 40.4322 - mae: 40.4322
Epoch 43/100
5/5 [==============================] - 0s 3ms/step - loss: 40.5367 - mae: 40.5367
Epoch 44/100
5/5 [==============================] - 0s 3ms/step - loss: 40.2487 - mae: 40.2487
Epoch 45/100
5/5 [==============================] - 0s 4ms/step - loss: 40.5152 - mae: 40.5152
Epoch 46/100
5/5 [==============================] - 0s 3ms/step - loss: 40.3702 - mae: 40.3702
Epoch 47/100
5/5 [==============================] - 0s 4ms/step - loss: 40.4769 - mae: 40.4769
Epoch 48/100
5/5 [==============================] - 0s 3ms/step - loss: 40.1532 - mae: 40.1532
Epoch 49/100
5/5 [==============================] - 0s 4ms/step - loss: 40.7291 - mae: 40.7291
Epoch 50/100
5/5 [==============================] - 0s 5ms/step - loss: 40.1536 - mae: 40.1536
Epoch 51/100
5/5 [==============================] - 0s 4ms/step - loss: 40.2711 - mae: 40.2711
Epoch 52/100
5/5 [==============================] - 0s 3ms/step - loss: 40.6572 - mae: 40.6572
Epoch 53/100
5/5 [==============================] - 0s 3ms/step - loss: 40.6573 - mae: 40.6573
Epoch 54/100
5/5 [==============================] - 0s 3ms/step - loss: 40.6894 - mae: 40.6894
Epoch 55/100
5/5 [==============================] - 0s 4ms/step - loss: 41.2771 - mae: 41.2771
Epoch 56/100
5/5 [==============================] - 0s 5ms/step - loss: 41.8519 - mae: 41.8519
Epoch 57/100
5/5 [==============================] - 0s 4ms/step - loss: 40.7903 - mae: 40.7903
Epoch 58/100
5/5 [==============================] - 0s 4ms/step - loss: 40.3128 - mae: 40.3128
Epoch 59/100
5/5 [==============================] - 0s 3ms/step - loss: 40.7198 - mae: 40.7198
Epoch 60/100
5/5 [==============================] - 0s 4ms/step - loss: 40.1478 - mae: 40.1478
Epoch 61/100
5/5 [==============================] - 0s 4ms/step - loss: 40.1116 - mae: 40.1116
Epoch 62/100
5/5 [==============================] - 0s 4ms/step - loss: 40.7800 - mae: 40.7800
Epoch 63/100
5/5 [==============================] - 0s 5ms/step - loss: 39.7242 - mae: 39.7242
Epoch 64/100
5/5 [==============================] - 0s 4ms/step - loss: 40.1465 - mae: 40.1465
Epoch 65/100
5/5 [==============================] - 0s 4ms/step - loss: 39.6887 - mae: 39.6887
Epoch 66/100
5/5 [==============================] - 0s 4ms/step - loss: 40.2840 - mae: 40.2840
Epoch 67/100
5/5 [==============================] - 0s 4ms/step - loss: 39.5541 - mae: 39.5541
Epoch 68/100
5/5 [==============================] - 0s 4ms/step - loss: 39.7378 - mae: 39.7378
Epoch 69/100
5/5 [==============================] - 0s 3ms/step - loss: 39.9784 - mae: 39.9784
Epoch 70/100
5/5 [==============================] - 0s 3ms/step - loss: 40.0016 - mae: 40.0016
Epoch 71/100
5/5 [==============================] - 0s 3ms/step - loss: 40.0913 - mae: 40.0913
Epoch 72/100
5/5 [==============================] - 0s 4ms/step - loss: 39.2547 - mae: 39.2547
Epoch 73/100
5/5 [==============================] - 0s 4ms/step - loss: 39.6828 - mae: 39.6828
Epoch 74/100
5/5 [==============================] - 0s 3ms/step - loss: 39.5373 - mae: 39.5373
Epoch 75/100
5/5 [==============================] - 0s 3ms/step - loss: 39.6265 - mae: 39.6265
Epoch 76/100
5/5 [==============================] - 0s 4ms/step - loss: 39.3110 - mae: 39.3110
Epoch 77/100
5/5 [==============================] - 0s 4ms/step - loss: 39.1599 - mae: 39.1599
Epoch 78/100
5/5 [==============================] - 0s 3ms/step - loss: 39.7550 - mae: 39.7550
Epoch 79/100
5/5 [==============================] - 0s 4ms/step - loss: 39.2542 - mae: 39.2542
Epoch 80/100
5/5 [==============================] - 0s 4ms/step - loss: 38.6968 - mae: 38.6968
Epoch 81/100
5/5 [==============================] - 0s 4ms/step - loss: 39.5442 - mae: 39.5442
Epoch 82/100
5/5 [==============================] - 0s 4ms/step - loss: 39.8686 - mae: 39.8686
Epoch 83/100
5/5 [==============================] - 0s 3ms/step - loss: 39.1693 - mae: 39.1693
Epoch 84/100
5/5 [==============================] - 0s 3ms/step - loss: 38.8840 - mae: 38.8840
Epoch 85/100
5/5 [==============================] - 0s 4ms/step - loss: 38.8887 - mae: 38.8887
Epoch 86/100
5/5 [==============================] - 0s 4ms/step - loss: 38.6614 - mae: 38.6614
Epoch 87/100
5/5 [==============================] - 0s 4ms/step - loss: 38.8399 - mae: 38.8399
Epoch 88/100
5/5 [==============================] - 0s 4ms/step - loss: 38.6604 - mae: 38.6604
Epoch 89/100
5/5 [==============================] - 0s 3ms/step - loss: 38.7559 - mae: 38.7559
Epoch 90/100
5/5 [==============================] - 0s 4ms/step - loss: 38.5442 - mae: 38.5442
Epoch 91/100
5/5 [==============================] - 0s 4ms/step - loss: 38.3247 - mae: 38.3247
Epoch 92/100
5/5 [==============================] - 0s 5ms/step - loss: 38.8431 - mae: 38.8431
Epoch 93/100
5/5 [==============================] - 0s 4ms/step - loss: 39.1137 - mae: 39.1137
Epoch 94/100
5/5 [==============================] - 0s 4ms/step - loss: 38.1463 - mae: 38.1463
Epoch 95/100
5/5 [==============================] - 0s 4ms/step - loss: 38.3998 - mae: 38.3998
Epoch 96/100
5/5 [==============================] - 0s 4ms/step - loss: 38.5599 - mae: 38.5599
Epoch 97/100
5/5 [==============================] - 0s 3ms/step - loss: 38.1038 - mae: 38.1038
Epoch 98/100
5/5 [==============================] - 0s 5ms/step - loss: 39.0081 - mae: 39.0081
Epoch 99/100
5/5 [==============================] - 0s 4ms/step - loss: 38.3056 - mae: 38.3056
Epoch 100/100
5/5 [==============================] - 0s 5ms/step - loss: 37.9976 - mae: 37.9976
<keras.callbacks.History at 0x7f59c89dbb50>
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.