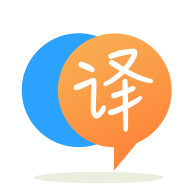
[英]getting TypeError: 'numpy.ndarray' object is not callable
[英]getting both “'numpy.ndarray' object is not callable” and “'Tensor' object is not callable”
我正在構建二進制分類器:我對ML沒有經驗,因此,使用從TensorFlow.org上的Iris分類教程改編的代碼,我在測試集上獲得了85%的准確性。 但是,此評估是使用0.5的閾值進行的:我希望能夠嘗試不同的閾值,只是為了看看我是否可以獲得更好的准確性。 所以我進入tensorflow網站並找到以下命令:
tf.metrics.precision_at_thresholds(
labels,
predictions,
thresholds,
weights=None,
metrics_collections=None,
updates_collections=None,
name=None
)
看起來就像我需要的,因為它可以讓我使用所需的任何自定義閾值來評估准確性。 因此,我將此代碼添加到了模型代碼中,最終結果看起來像這樣:
from __future__ import absolute_import
from __future__ import division
from __future__ import print_function
import numpy as np
import tensorflow as tf
train_file = "/home/javier/train.csv"
test_file = "/home/javier/test.csv"
def main():
# Load datasets.
training_set = tf.contrib.learn.datasets.base.load_csv_with_header(
filename=train_file,
target_dtype=np.int,
features_dtype=np.float32)
test_set = tf.contrib.learn.datasets.base.load_csv_with_header(
filename=test_file,
target_dtype=np.int,
features_dtype=np.float32)
# Specify that all features have real-value data
feature_columns = [tf.contrib.layers.real_valued_column("", dimension=15)]
# Build 3 layer DNN with 10, 20, 10 units respectively.
classifier = tf.contrib.learn.DNNClassifier(feature_columns=feature_columns,
hidden_units=[15,20,15],
optimizer=tf.train.ProximalAdagradOptimizer(learning_rate=0.05,l2_regularization_strength=0.2),
n_classes=2,
model_dir="/home/javier/tf_tinkering")
# Define the training inputs
def get_train_inputs():
x = tf.constant(training_set.data)
y = tf.constant(training_set.target)
return x, y
# Fit model.
classifier.fit(input_fn=get_train_inputs, steps=50)
# Define the test inputs
def get_test_inputs():
x = tf.constant(test_set.data)
y = tf.constant(test_set.target)
return x, y
# Evaluate accuracy.
tf.metrics.precision_at_thresholds(
tf.constant(test_set.target),
classifier.predict(input_fn=tf.constant(test_set.data)),
thresholds=[0.5,0.4,0.6],
)
if __name__ == "__main__":
main()
問題在於tf.metrics無法解釋“預測”位。 我嘗試了不同的調用“預測”的方法,它們都返回錯誤。 運用
tf.metrics.precision_at_thresholds(
tf.constant(test_set.target),
classifier.predict_classes(input_fn=get_test_inputs),
thresholds=[0.5,0.4,0.6],
)
給我
TypeError: Expected binary or unicode string, got <generator object <genexpr> at 0x7ff38d2c5af0>
運用
tf.metrics.precision_at_thresholds(
tf.constant(test_set.target),
classifier.predict_classes(input_fn=tf.constant(test_set.data)),
thresholds=[0.5,0.4,0.6],
)
結果是
TypeError: 'Tensor' object is not callable
和使用
tf.metrics.precision_at_thresholds(
tf.constant(test_set.target),
classifier.predict_classes(input_fn=test_set.data),
thresholds=[0.5,0.4,0.6],
)
輸出
TypeError: 'numpy.ndarray' object is not callable
我什至嘗試定義一個新的矩陣“ feature_columns_matrix”,然后將其從csv文件中粘貼到所有值中,然后運行“ classifier.predict_classes(input_fn = feature_columns_matrix)”,該矩陣也無法正常工作。 在測試集上運行到tf.metrics子例程時,如何傳遞網絡輸出層的值?
我已經在該網站上閱讀了大約10個其他類似問題,但沒有一個對我有幫助(只是您知道我不是在問一個多余的問題)。 任何幫助將不勝感激! 謝謝
更新:我發現正在運行
print(list(classifier.predict(input_fn=get_test_inputs)))
正確返回測試文件中每個樣本的預測類。 但是,這不是我為tf.metrics評估准確性所需要的,因為上述命令正是基於0.5閾值返回類! 它沒有給出網絡最后一層的實際輸出。 當我運行上面的命令時,我得到了:
[0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0]
但是我真正需要的是當網絡在測試集上運行時產生的實際float32值。 這樣,我可以將其輸入tf.metrics並測試不同的閾值。 有人知道該怎么做嗎?
TypeError: 'numpy.ndarray' object is not callable
這意味着您此時有一個numpy數組,並且您試圖將其當作函數使用。 也就是說,您正在使用arr(...)
語法“調用”它。 您要么應該為arr[...]
為其建立索引,要么該對象首先不應該是數組。
與張量對象相同。
TypeError: Expected binary or unicode string, got <generator object <genexpr> at 0x7ff38d2c5af0>
表示該函數希望將字符串作為參數,但是您給了它其他東西( generator
的概念可能對您而言太高級了。)
理想情況下,使用Python進行編程時,您需要了解每個變量所引用的對象,尤其是哪種對象。 是一個函數,一個字符串,一個數字,一個數組和tensorflow對象。 當您遇到此類錯誤時,您需要添加診斷打印來檢查對象。 不要只是假設; 測試。
顯然,您在此表達式中嘗試不同的事情:
classifier.predict_classes(input_fn=get_test_inputs)
classifier.predict_classes(input_fn=tf.constant(test_set.data))
classifier.predict_classes(input_fn=test_set.data)
此功能接受什么? 我猜test_set.dat
是一個numpy數組。 將其包裝在tf.constant()
其轉變為張量對象。 而get_test_inputs
是一個生成器/函數。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.