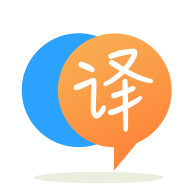
[英]Keras multivariate time series forecasting model returns NaN as MAE and loss
[英]Multivariate time series forecasting with LSTMs in Keras (on future data)
所以我一直在使用 Keras 來預測多元時間序列。 該數據集是污染數據集。 第一列是我要預測的內容,其余 7 列是特征。 數據集可以在這里找到: https://github.com/sagarmk/Forecasting-on-Air-pollution-with-RNN-LSTM/blob/master/pollution.csv
所以我想做的是在沒有“污染”列的測試集上執行以下代碼。 假設有新的特征數據,但沒有污染。 所以
為了簡單起見,最初可以將數據集拆分為訓練和測試數據集,從測試數據集中刪除“污染”列?
請參閱下面的簡單代碼。 這里我只是簡單地導入和處理數據集。
import numpy as np
import pandas as pd
import matplotlib as plt
import seaborn as sns
import plotly.express as px
# Import data
dataset = pd.read_csv("pollution.csv")
dataset = dataset.drop(['date'], axis = 1)
label, layer = pd.factorize(dataset['wnd_dir'])
dataset['wnd_dir'] = pd.DataFrame(label)
dataset=dataset.fillna(dataset.mean())
dataset.head()
之后,我對數據集進行規范化。
from sklearn.preprocessing import MinMaxScaler
values = dataset.values
scaler = MinMaxScaler()
scaled = scaler.fit_transform(values)
scaled[0]
然后將歸一化的數據轉換為監督形式。
def to_supervised(dataset,dropNa = True,lag = 1):
df = pd.DataFrame(dataset)
column = []
column.append(df)
for i in range(1,lag+1):
column.append(df.shift(-i))
df = pd.concat(column,axis=1)
df.dropna(inplace = True)
features = dataset.shape[1]
df = df.values
supervised_data = df[:,:features*lag]
supervised_data = np.column_stack( [supervised_data, df[:,features*lag]])
return supervised_data
timeSteps = 2
supervised = to_supervised(scaled,lag=timeSteps)
pd.DataFrame(supervised).head()
現在數據集被分割和轉換,以便 LSTM 網絡可以處理它。
features = dataset.shape[1]
train_hours = round(dataset.shape[0]*0.7)
X = supervised[:,:features*timeSteps]
y = supervised[:,features*timeSteps]
x_train = X[:train_hours,:]
x_test = X[train_hours:,:]
y_train = y[:train_hours]
y_test = y[train_hours:]
print(x_train.shape,x_test.shape,y_train.shape,y_test.shape)
#convert data to fit for lstm
#dimensions = (sample, timeSteps here it is 1, features )
x_train = x_train.reshape(x_train.shape[0], timeSteps, features)
x_test = x_test.reshape(x_test.shape[0], timeSteps, features)
print(x_train.shape,x_test.shape)
這里訓練了 model
#define the model
from keras.models import Sequential
from keras.layers import Dense,LSTM
model = Sequential()
model.add( LSTM( 50, input_shape = ( timeSteps,x_train.shape[2]) ) )
model.add( Dense(1) )
model.compile( loss = "mae", optimizer = "adam")
history = model.fit( x_train,y_train, validation_data = (x_test,y_test), epochs = 50 , batch_size = 72, verbose = 0, shuffle = False)
plt.pyplot.plot(history.history['loss'], label='train')
plt.pyplot.plot(history.history['val_loss'], label='test')
plt.pyplot.legend()
#plt.pyplot.yticks([])
#plt.pyplot.xticks([])
plt.pyplot.title("loss during training")
plt.pyplot.show()
最后我 plot 訓練數據和測試數據。
y_pred = model.predict(x_test)
x_test = x_test.reshape(x_test.shape[0],x_test.shape[2]*x_test.shape[1])
inv_new = np.concatenate( (y_pred, x_test[:,-7:] ) , axis =1)
inv_new = scaler.inverse_transform(inv_new)
final_pred = inv_new[:,0]
plt.pyplot.figure(figsize=(20,10))
plt.pyplot.plot(dataset['pollution'])
plt.pyplot.plot([None for i in dataset['pollution']] + [x for x in final_pred])
plt.pyplot.show()
使用您的神經網絡預測結果應該像下面的代碼行一樣簡單。 傳遞與訓練數據格式相同的新數據。
prediction = model.predict(features)
你有任何可以提供的代碼嗎? 你遇到了什么問題?
即,當“測試”數據集僅包含 8 個特征列而沒有價格列時?
看起來你在問一個特征工程問題。 你真正的數據集在不同的列中有 nan 值,這使得預測失敗,對吧?
在這種情況下,您可以采取常見的解決方案:
用 trainset 中對應列的中值/平均值填充 nan 值。
例如,您可以通過每種產品最近 14 天(聚合長度)價格的中位數/平均值來填充未來價格。
在進行未來預測時,可能有很多特征只有歷史(沒有計划)。 在傳統的機器學習中,如果你想預測一個依賴於所有特征的目標,你需要先預測這些特征的未來。
但是通過 LSTM,您可以一並進行預測,檢查time_series#multi-output_models
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.