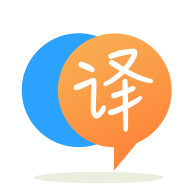
[英]Unity breakout game change direction of ball based on which part of the paddle it made with collision using c#
[英]How to respawn a paddle and ball in breakout - Unity 3D C#
我正在 3D 中制作一款突破性游戲,這是我第一次制作游戲並使用 Unity,所以我有點無能為力。 我已經達到了我的游戲正常運行的地步,直到球離開屏幕並進入“死區”。 有人可以建議如何一起重生槳和球並繼續游戲嗎? 我在下面包含了我的球和槳腳本,我也有一個用於積木的腳本,但不確定是否相關。 我還制作了一個球和槳的預制件,但不知道如何處理它。 感謝任何能提供幫助的人:)
我的球的代碼
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class BallScript : MonoBehaviour
{
public Rigidbody rbody;
public float MinVertMovement = 0.1f;
public float MinSpeed = 10f;
public float MaxSpeed = 10f;
private bool hasBeenLaunched = false;
void Start()
{
}
private float minVelocity = 10f;
private Vector3 lastFrameVelocity;
void FixedUpdate()
{
if (hasBeenLaunched == false)
{
if (Input.GetKey(KeyCode.Space))
{
Launch();
}
}
if (hasBeenLaunched)
{
Vector3 direction = rbody.velocity;
direction = direction.normalized;
float speed = direction.magnitude;
if (direction.y>-MinVertMovement && direction.y <MinVertMovement)
{
direction.y = direction.y < 0 ? -MinVertMovement : MinVertMovement;
direction.x = direction.x < 0 ? -1 + MinVertMovement : 1 - MinVertMovement;
rbody.velocity = direction * MinSpeed;
}
if (speed<MinSpeed || speed>MaxSpeed)
{
speed = Mathf.Clamp(speed, MinSpeed, MaxSpeed);
rbody.velocity = direction*speed;
}
}
lastFrameVelocity = rbody.velocity;
}
void OnCollisionEnter(Collision collision)
{
Bounce(collision.contacts[0].normal);
}
private void Bounce(Vector3 collisionNormal)
{
var speed = lastFrameVelocity.magnitude;
var direction = Vector3.Reflect(lastFrameVelocity.normalized, collisionNormal);
Debug.Log("Out Direction: " + direction);
rbody.velocity = direction * Mathf.Max(speed, minVelocity);
}
public void Launch()
{
rbody = GetComponent<Rigidbody>();
Vector3 randomDirection = new Vector3(-5f, 10f, 0);
randomDirection = randomDirection.normalized * MinSpeed;
rbody.velocity = randomDirection;
transform.parent = null;
hasBeenLaunched = true;
}
}
我的槳的代碼
public class PaddleScript : MonoBehaviour
{
private float moveSpeed = 15f;
void Start()
{
}
void Update()
{
if (Input.GetKey(KeyCode.RightArrow) && transform.position.x<9.5)
transform.Translate(moveSpeed *Input.GetAxis("Horizontal")*Time.deltaTime, 0f, 0f);
if (Input.GetKey(KeyCode.LeftArrow) && transform.position.x>-7.5)
transform.Translate(moveSpeed *Input.GetAxis("Horizontal")*Time.deltaTime, 0f, 0f);
}
}
檢查球是否離開屏幕的最簡單的方法是立即在相機周邊放置一個觸發器,並為你的球添加一個OnTriggerEnter2D方法。
/* Inside the ball script */
private void OnTriggerEnter() { // Use the 2D version if you're using 2D colliders
/* Respawning stuff */
}
由於您可能希望在觸發該方法時發生一堆不同的事情,因此您可能希望使用Unity Event ,這不是性能之王,但對於像breakout這樣的游戲來說可能並不重要。
using UnityEngine.Events;
public class BallScript : MonoBehaviour
{
public UnityEvent onBallOut;
/* ... */
private void OnTriggerEnter() {
onBallOut.Invoke();
}
}
然后,您可能需要一個 Respawn() 方法(不是 Reset(),因為這是默認的 MonoBehaviour 調用),它將球放回其原始 position,您可以在場景加載后立即將其存儲在字段中:
private Vector3 defaultPosition;
private void Start() {
defaultPosition = transform.position;
}
PS:如果你沒有在你的 paddle 腳本中使用 Start() 方法,那么刪除它,因為它會被 Unity 調用,即使它是空的。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.